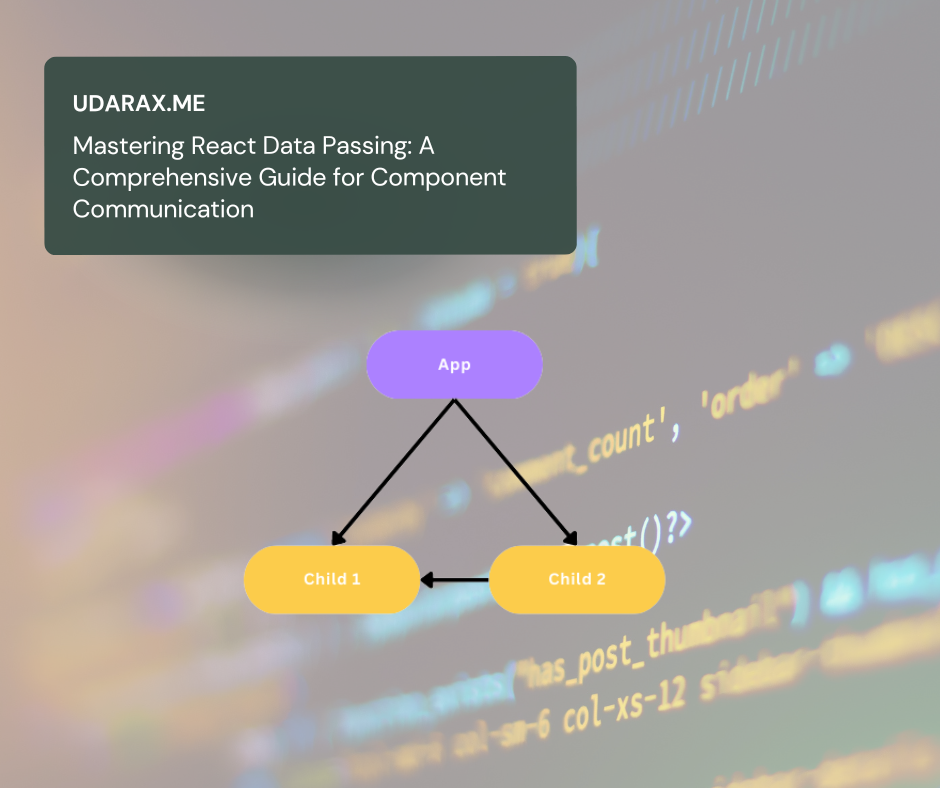
In this article we are going to talk about react data passing. Parsing data can help to keep the data consistent and predictable across the application, and make it easier to manage and update. To pass data you can use several techniques like use callbacks, events, or a state management library like Redux.
1. Introduction to React Data Passing
There are 3 main types for passing data between react components.
- Parent to child (using props)
- Child to parent (using callbacks)
- Child to child ( between siblings using callbacks then props )
App (Parent)
└── Component
└── Child1.js (Child1)
└── Child2.js (Child2)
2. React data passing from Parent to child using props.
App (Parent)
└── Component/Child1.js (Child1)
import React , { useState } from 'react'
import Child1 from './Component/Child1';
function App() {
return (
<div className="App">
<div>Parent</div>
<Child1 passData = {'This data from Parent'} />
</div>
)
}
export default App
import React from 'react'
export default function Child1({passData}) {
return (
<div>
<div>Child1</div>
<p>{passData}</p>
</div>
)
}
3. React data passing from Child to parent using callbacks.
App (Parent)
└── Component/Child1.js (Child1)
First you need to import your child component into the parent component. Then you can add a poprs and you can name it whatever you need. In my case I used ‘passData’ as my props name.
import React , { useState } from 'react'
import Child1 from './Component/Child1';
function App() {
const [passData, setPassData] = useState('');
const getData = (message) => {
setPassData(message);
};
return (
<div className="App">
<div>This data from Child1 : {passData}</div>
<Child1 getData = {getData} />
</div>
)
}
export default App
import React from "react";
export default function Child1({ getData }) {
return (
<div>
<div>Child1</div>
<button onClick={() => getData("This is test message")}>Send Data</button>
</div>
);
}
So, on click of the getData button ‘This is test message’ will now be passed to the ‘getData’ handler function in the Parent component and the new value of data in the Parent component will now be ‘This is test message’. This way, data from the child component(data in the variable msg in Child component) is passed to the parent component.
4. React data passing from Child to another child ( between siblings using callbacks then props )
App (Parent)
└── Component
└── Child1.js (Child1)
└── Child2.js (Child2)
import React , { useState } from 'react'
import Child1 from './Component/Child1';
import Child2 from './Component/Child2';
function App() {
const [passData, setPassData] = useState('');
return (
<div className="App">
<Child1 passData={passData} />
<Child2 setPassData={setPassData}/>
</div>
)
}
export default App
import React from "react";
return (
<div>
<div>Child1 data : {passData} </div>
</div>
);
}
import React from "react";
export default function Child2({ setPassData }) {
return (
<div>
<button onClick={() => setPassData("This is from child2")}>
Send Data
</button>
</div>
);
}