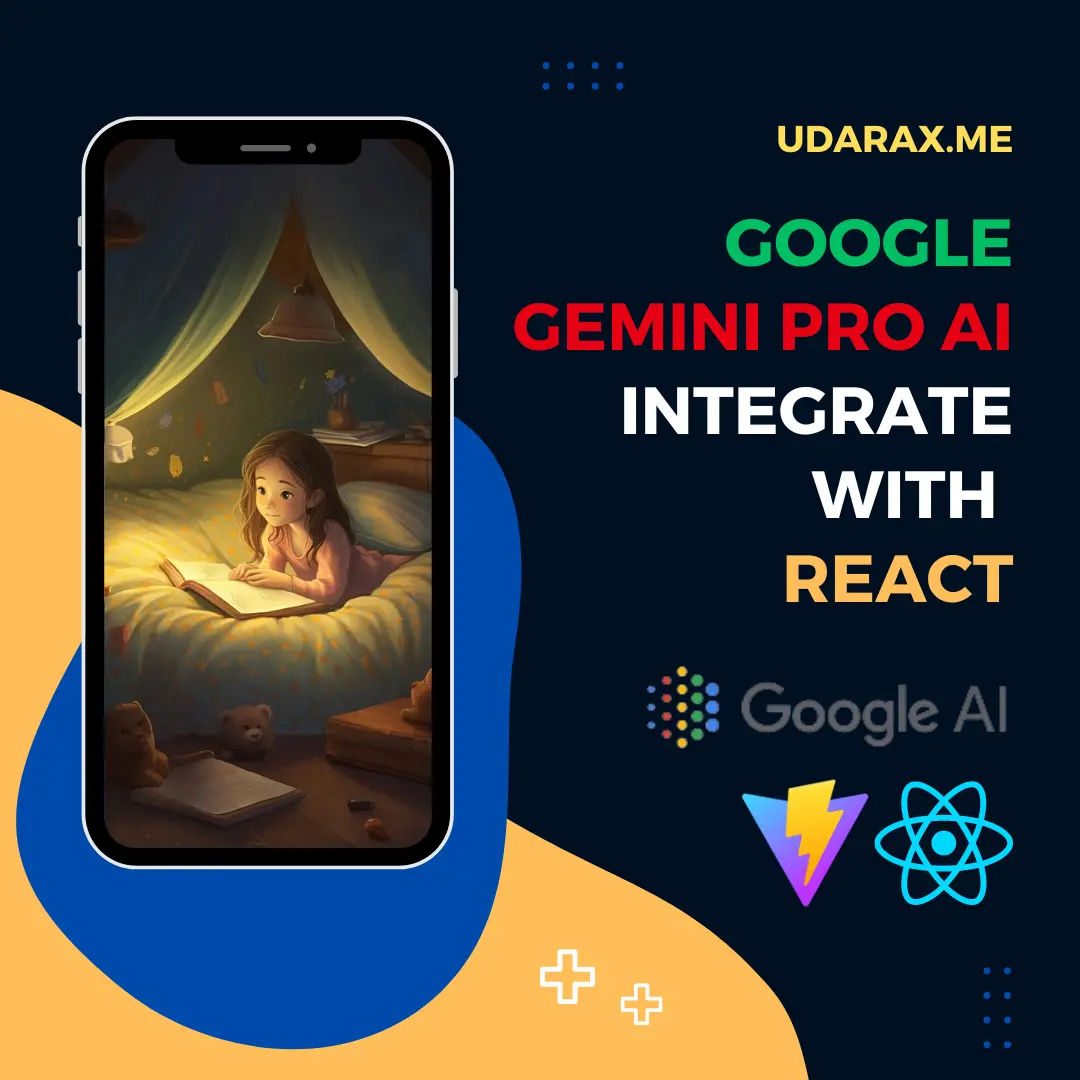
Are you a budding developer eager to explore the world of AI and React? In this tutorial, we’ll guide you through creating a bedtime story generator web app using Google Gemini Pro AI and Vite React. This beginner-friendly project combines the power of AI storytelling with the simplicity of React, providing a delightful experience for users.
Demo Application :- https://bedtime-story-udarax.netlify.app/
1.Introduction
Unlock the world of AI and React development with our comprehensive tutorial on building a captivating Bedtime Story Generator web app using Google Gemini Pro AI and Vite React. Ideal for beginners, this hands-on guide explores the seamless integration of cutting-edge AI technology, making coding accessible and enjoyable. Dive into trending topics such as AI storytelling, web development, and creative coding. Discover the power of Google Gemini Pro API, create personalized bedtime stories for children, and elevate your coding skills. Follow our step-by-step instructions to embark on a journey that blends education, creativity, and the latest in technology.
2. Prerequisites
Before we dive in, make sure you have the following:
- Google Gemini Pro API Key: Obtain your API key by signing up on Google Maker Suite. Note that while you may need to set up billing for the API key to work, Google Gemini Pro AI offers free usage.
- React Development Environment: We’ll use Vite React for our frontend. Ensure Node.js is installed, and then set up your React project using Vite.
3. Setting Up the React App
Now, let’s guide you through setting up a project.
3.1 Create Your React App
Open your terminal inside the working folder and run below command :
npm create vite@latest
cd gemini-pro
npm install
3.2 Install Dependencies
npm install @google/generative-ai
3.3 Add Bootstrap
Replace Index.html file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Vite + React</title>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin="anonymous"
/>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"
integrity="sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin="anonymous"
></script>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
<script
src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.11.6/dist/umd/popper.min.js"
integrity="sha384-oBqDVmMz9ATKxIep9tiCxS/Z9fNfEXiDAYTujMAeBAsjFuCZSmKbSSUnQlmh/jp3"
crossorigin="anonymous"
></script>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.min.js"
integrity="sha384-cuYeSxntonz0PPNlHhBs68uyIAVpIIOZZ5JqeqvYYIcEL727kskC66kF92t6Xl2V"
crossorigin="anonymous"
></script>
</body>
</html>
4. Exploring the Code
Let’s dive into the key components of the code:
4.1 Replace App.jsx File
import { useState } from "react";
import "./App.css";
import { GoogleGenerativeAI } from "@google/generative-ai";
function App() {
const [loading, setLoading] = useState(false);
const [apiData, setApiData] = useState([]);
const [name, setName] = useState("");
const [gender, setGender] = useState("");
const [age, setAge] = useState("");
const [country, setCountry] = useState("");
const [hobbies, setHobbies] = useState("");
const genAI = new GoogleGenerativeAI(
"YOUR-API-KEY-HERE"
);
const fetchData = async () => {
const model = genAI.getGenerativeModel({ model: "gemini-pro" });
const prompt = `Think you are a mom who has ${age} years old ${gender} in ${country}. your child's favourite things are ${hobbies}.You are a caring mother about your
children and you have to tell a wonderful story for your child every single night. so generate a bedtime story for the chield bease on child's favourite things, country, age.
also end of the story you have to give some advice for kids to be good and do good for the sociaty. Also motivate them to belive themselefs`;
const result = await model.generateContent(prompt);
const response = await result.response;
const text = response.text();
setApiData(text);
setLoading(false);
};
const handleSubmit = (e) => {
e.preventDefault();
setLoading(true);
console.log(name, gender, age , country, hobbies);
fetchData();
};
return (
<div className="container">
<h1>Google Gemini Pro AI Integration With React</h1>
<div className="mt-5 mb-5">
<form onSubmit={handleSubmit}>
<div className="row d-flex align-items-end">
<div className="col-lg-2">
<label htmlFor="name" className="form-label">
Name
</label>
<input
type="text"
className="form-control"
id="name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div className="col-lg-2">
<label htmlFor="gender" className="form-label">
Gender
</label>
<select
className="form-select"
id="gender"
value={gender}
onChange={(e) => setGender(e.target.value)}
>
<option value="">Select Gender</option>
<option value="boy">Boy</option>
<option value="Girl">Girl</option>
</select>
</div>
<div className="col-lg-2">
<label htmlFor="age" className="form-label">
Age
</label>
<input
type="text"
className="form-control"
id="age"
value={age}
onChange={(e) => setAge(e.target.value)}
/>
</div>
<div className="col-lg-2">
<label htmlFor="country" className="form-label">
Country
</label>
<input
type="text"
className="form-control"
id="country"
value={country}
onChange={(e) => setCountry(e.target.value)}
/>
</div>
<div className="col-lg-2">
<label htmlFor="hobbies" className="form-label">
Hobbies
</label>
<input
type="text"
className="form-control"
id="hobbies"
value={hobbies}
onChange={(e) => setHobbies(e.target.value)}
/>
</div>
<div className="col-lg-2">
<button type="submit" className="btn btn-primary mt-3 col-lg-12">
Submit
</button>
</div>
</div>
</form>
</div>
<div className="">
{!loading && <p className="text-align-left">{apiData}</p>}
{loading && <p>Loading...</p>}
</div>
<div className="mt-4">
Developed By <a href="https://udarax.me">UDARAX</a>
</div>
</div>
);
}
export default App;
4.2 Add Google Gemini Pro API Key
Replace “YOUR-API-KEY-HERE” in the GoogleGenerativeAI instantiation with your actual API key.
4.3 Fetch Data Function
const fetchData = async () => {
const model = genAI.getGenerativeModel({ model: "gemini-pro" });
const prompt = `Think you are a mom who has ${age} years old ${gender} in ${country}. your child's favourite things are ${hobbies}.You are a caring mother about your
children and you have to tell a wonderful story for your child every single night. so generate a bedtime story for the chield bease on child's favourite things, country, age.
also end of the story you have to give some advice for kids to be good and do good for the sociaty. Also motivate them to belive themselefs`;
const result = await model.generateContent(prompt);
const response = await result.response;
const text = response.text();
setApiData(text);
setLoading(false);
};
4.4 Form Handling
Add the following function in your App.jsx to handle form submission:
const handleSubmit = (e) => {
e.preventDefault();
setLoading(true);
fetchData();
This form handles user input, triggers data fetching, and sets the loading state.
<form onSubmit={handleSubmit}>
{/* ... (Omitted for brevity) */}
<button type="submit" className="btn btn-primary mt-3 col-lg-12">
Submit
</button>
</form>
4.5 Display Result
<div>
{!loading && <p className="text-align-left">{apiData}</p>}
{loading && <p>Loading...</p>}
</div>
5. Conclusion
Congratulations! You’ve successfully built a bedtime story generator web app using Google Gemini Pro AI and React. This project not only introduces you to the exciting world of AI but also enhances your React skills.
Feel free to explore and customize the app further. You can add more features, improve the UI, or even integrate additional AI capabilities. Happy coding!
Additional Resources
Google Generative AI Documentation For Node JS :- https://ai.google.dev/tutorials/node_quickstart