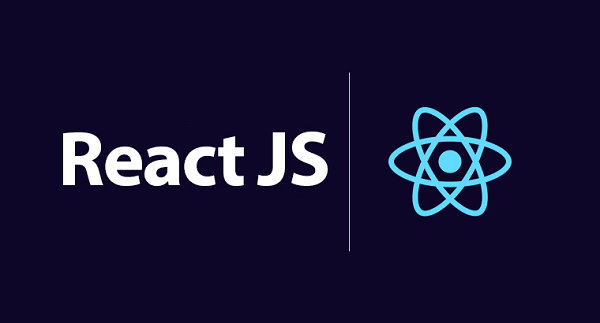
Requirements you need.
- React Js
- Axios
- Bootstrap
Create React App
The first step is in this tutorial is install react app. And you can do this using NPM.
npx create-react-app my-app
Now, Open the project in your code editor once the project is created.
Install Axios
In this tutorial I am going to use Axios for fetch the data from APIs . So now we are going to install it.
Using NPM
npm install axios
Using Yarn
yarn add axios
Axios Usage
There are many ways to get data from a API in React Js. But using Axios it is very easy. You just need to follow below code to fetch data.
axios({
method: 'get',
url: 'http://ANY_API-URL',
})
.then(function (response) {
console.log(response)
});
Create a Component
To simplify the process, I created a child component to display the relevant data. To do that first, you need to create a folder called “component” in the src directory. Then inside that directory create a new file called box.js
box.js
import React from 'react'
export default function box({count,name}) {
return (
<div className="col-lg-3 ">
<div className="box">
<div>{count}</div>
<div>{name}</div>
</div>
</div>
)
}
Now we are going to add bootstrap CDN into the index.html file. that file is located in the public folder. Open the index.html file and copy and past below link and script tags in the head tag
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-0evHe/X+R7YkIZDRvuzKMRqM+OrBnVFBL6DOitfPri4tjfHxaWutUpFmBp4vmVor"
crossorigin="anonymous"
/>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/js/bootstrap.bundle.min.js"
integrity="sha384-pprn3073KE6tl6bjs2QrFaJGz5/SUsLqktiwsUTF55Jfv3qYSDhgCecCxMW52nD2"
crossorigin="anonymous"
></script>
Now open the app.css file and remove every styles and past below styles into that file. app.css is located in the src folder.
* {
margin: 0;
padding: 0;
}
.titleMain{
color: #fff;
text-align: center;
}
.contentBox{
padding: 50px;
}
.box{
color: #fff;
height: 200px;
border: 1px solid #F24843;
border-radius: 15px;
margin-bottom: 20px;
padding: 20px;
}
It’s time to create App.js file. You can make it clean and then past below code into App.js file. I used useEffect react hook to call the fetch data function.
import axios from "axios";
import React, { useEffect, useState } from "react";
import "./App.css";
import Box from './component/box'
function App() {
const [apiData, setApiData] = useState();
const [loading, setLoading] = useState(true);
const fecthData = async () => {
axios
.get("https://www.hpb.health.gov.lk/api/get-current-statistical")
.then(function (response) {
setApiData(response.data.data);
setLoading(false);
console.log(response.data.data);
})
.catch(function (error) {
console.log(error);
});
};
useEffect(() => {
fecthData();
}, []);
return (
<div className="container">
<h1 className="titleMain">Covid-19 Dashboard - Sri Lanka</h1>
<h3 className="titleMain">{!loading && apiData.update_date_time}</h3>
<div className="contentBox">
<div className="row">
{!loading && (
<>
<Box count={apiData.global_deaths} name="Global Deaths"/>
<Box count={apiData.global_new_cases} name="Global New Cases"/>
<Box count={apiData.global_new_deaths} name="Global New Deaths"/>
<Box count={apiData.global_recovered} name="Global Recoverd"/>
<Box count={apiData.local_active_cases} name="Local Active Cases"/>
<Box count={apiData.local_deaths} name="Local Deaths"/>
<Box count={apiData.local_new_cases} name="Local New Cases"/>
<Box count={apiData.local_recovered} name="Local Recovered"/>
</>
)}
</div>
</div>
</div>
);
}
export default App;
blow is the output you should get after following all the steps given by me. If you have any issue with this tutorial contact me in the contact page. I will help you to find out the errors. And to run the project, just type below command in your terminal or CMD and hit enter button. Make sure you are in the root directory in your relevant project. Otherwise you will have an errors.
npm start