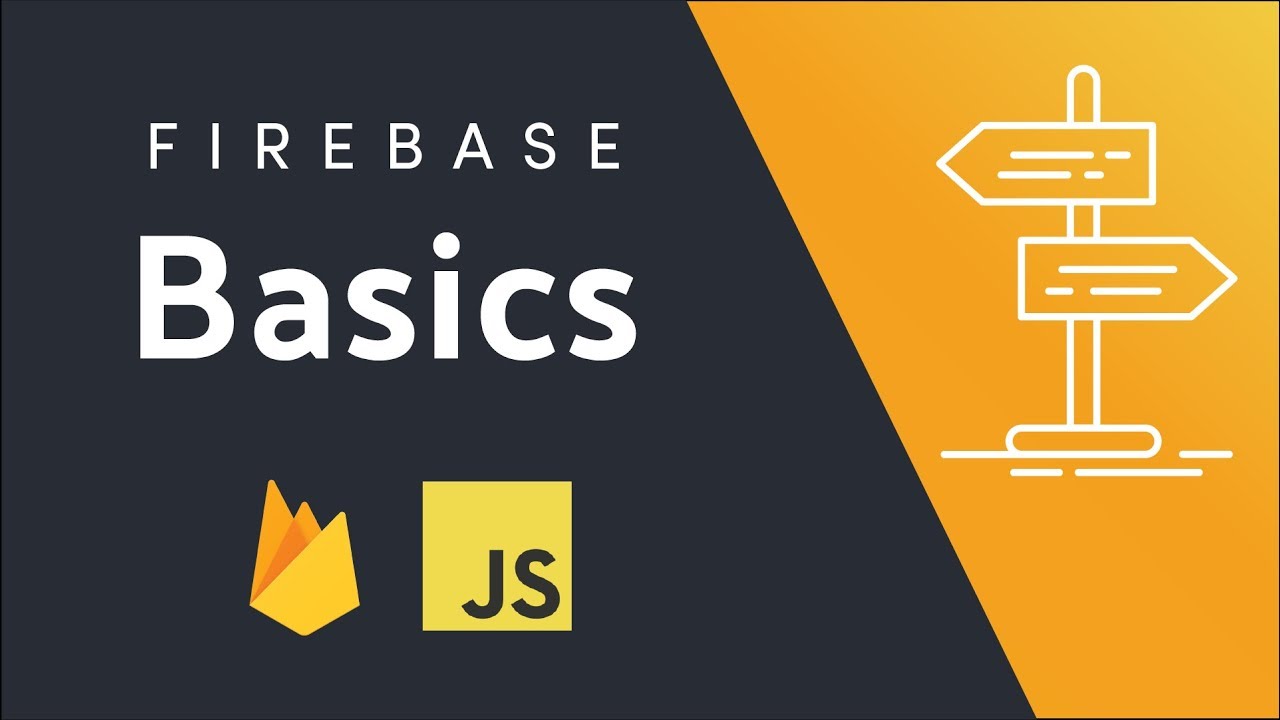
Requirements you need.
- Google Account
- Firebase Account
What is Firebase Real Time Database ?
The Firebase Realtime Database is a cloud-hosted NoSQL database that lets you store and sync data between your users in realtime.It is a cloud-hosted database. Data is stored as JSON and synchronized in realtime to every connected client. When you build cross-platform apps with our Apple platforms, Android, and JavaScript SDKs, all of your clients share one Realtime Database instance and automatically receive updates with the newest data.
In today we are going to build a read and write data application using Reatltime database with JavaScript.
Create a Firebase Account - Step 01
The first thing is to create this application, create an account. Click here to create an account.
Sign In with google for create an account. And then you will redirect automatically to recent project page. In your case it will be a empty page with no projects. Now is the time to create new Project. For that click on the add project box and create a project by given a name.
Then you will redirect automatically to the project overview page. In that page you have to do is add the platform. To do that click on the web button. Relevant image is attached below.
Register you app by given a name. And leave the Hosting check box as it is ( unhacked ). Because we are not going to use that feature for our application.
In the second step, you have to choose “use a<script> tag” option and copy the tag and we will use it for our application. That part is very important. Then click on continue to console button.
Create Realtime Database - Step 02
For create a Realtime database select the Build menu from the left side menu bar. Then click on the Realtime database.
After the page is loaded, Click on Create Database button. Then select your proffered datacenter from database options and click next. Then select Start in locked mode to create our database and click enable.
Next important part is change the database rules. To do that you have to navigate Rules section. You can find that top of the page.
Then change the read and write rules like below and publish it once you changed
Create Application - Step 03
We can use any framework to design frontend. But for this I just use bootstrap. And also use jQuery for some Js things.
Now create a new file called app.js and put the copied details of project in the step 02.
app.js
const firebaseConfig = {
apiKey: "",
authDomain: "",
projectId: "",
messagingSenderId: "",
appId: "",
measurementId: ""
};
// Initialize Firebase
const app = firebase.initializeApp(firebaseConfig);
var database = firebase.database();
Then create another new file and name it as index.html. Import all the scripts in the head section on the index.html file like below and I added some more styles for future works.
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css"
rel="stylesheet" />
<!-- JavaScript Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<script src="https://polyfill.io/v3/polyfill.min.js?features=default"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-analytics.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-auth.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-database.js"></script>
<title>Udarax.me Post 02</title>
<style>
.content{
padding: 50px;
}
.addsection , .viewSection{
margin-top: 50px;
}
</style>
</head>
Create body section to display data like below.
<div class="container">
<div class="content">
<h3>Read and Write Data with JavaScript</h3>
<div class="addsection">
<div class="row">
<div class="col-lg-3">
<div class="form-group">
<label for="">Name</label>
<input type="text" name="name" id="name" class="form-control">
</div>
</div>
<div class="col-lg-3">
<div class="form-group">
<label for="">Age</label>
<input type="text" name="age" id="age" class="form-control">
</div>
</div>
<div class="col-lg-4">
<div class="form-group">
<label for="">Address</label>
<input type="text" name="address" id="address" class="form-control">
</div>
</div>
<div class="col-lg-2">
<div class="form-group">
<label for="">Action</label>
<input type="button" name="addButon" id="addButon" class="btn btn-success col-lg-12" value="Add">
</div>
</div>
</div>
</div>
<div class="viewSection">
<table class="table" id="userTable">
<thead>
<tr>
<th scope="col">Name</th>
<th scope="col">Age</th>
<th scope="col">Address</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
</div>
</div>
Read Data
var ref = firebase.database().ref('/Users/');
ref.on("value", function (snapshot) {
const data = snapshot.val();
}, function (error) {
console.log("Error: " + error.code);
});
Write Data
firebase.database().ref('/Users/').push({
name: name,
age: age,
address : address
});
index.html (final edits)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<!-- CSS only -->
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css"
rel="stylesheet"
/>
<!-- JavaScript Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" ></script>
<script src="https://polyfill.io/v3/polyfill.min.js?features=default"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-analytics.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-auth.js"></script>
<script src="https://www.gstatic.com/firebasejs/7.13.2/firebase-database.js"></script>
<title>Udarax.me Post 02</title>
<style>
.content{
padding: 50px;
}
.addsection , .viewSection{
margin-top: 50px;
}
</style>
</head>
<body>
<div class="container">
<div class="content">
<h3>Read and Write Data with JavaScript</h3>
<div class="addsection">
<div class="row">
<div class="col-lg-3">
<div class="form-group">
<label for="">Name</label>
<input type="text" name="name" id="name" class="form-control">
</div>
</div>
<div class="col-lg-3">
<div class="form-group">
<label for="">Age</label>
<input type="text" name="age" id="age" class="form-control">
</div>
</div>
<div class="col-lg-4">
<div class="form-group">
<label for="">Address</label>
<input type="text" name="address" id="address" class="form-control">
</div>
</div>
<div class="col-lg-2">
<div class="form-group">
<label for="">Action</label>
<input type="button" name="addButon" id="addButon" class="btn btn-success col-lg-12" value="Add">
</div>
</div>
</div>
</div>
<div class="viewSection">
<table class="table" id="userTable">
<thead>
<tr>
<th scope="col">Name</th>
<th scope="col">Age</th>
<th scope="col">Address</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
</div>
</div>
<script type="module">
import { initializeApp } from "https://www.gstatic.com/firebasejs/9.8.1/firebase-app.js";
import { getAnalytics } from "https://www.gstatic.com/firebasejs/9.8.1/firebase-analytics.js";
</script>
<script src="app.js"></script>
<script>
function resetInputs(){
$("#name").val("");
$("#age").val("");
$("#address").val("");
}
var ref = firebase.database().ref('/Users/');
ref.on("value", function (snapshot) {
var result = [];
let arr = [];
const data = snapshot.val();
arr.push(data);
$("#userTable").find("tr:gt(0)").remove();
console.log("This");
console.log(result);
console.log(arr.length-1);
for(var i in arr[0]){
result.push([i, arr[0][i]]);
}
console.log(result);
for (i = 0; i <result.length; i++) {
let user_id = String(result[i][0]);
markup = "<tr class='trow'><td>" +
result[i][1]['name'] + "</td><td>" +
result[i][1]['age'] + "</td><td>" +
result[i][1]['address'] + "</td></tr>"
tableBody = $("#userTable tbody");
tableBody.append(markup);
}
}, function (error) {
console.log("Error: " + error.code);
});
function deleteUser(id){
console.log(id);
}
$("#addButon").click(function (){
console.log("Clicked");
$("#userTable").find("tr:gt(0)").remove();
let name = $("#name").val();
let age = $("#age").val();
let address = $("#address").val();
firebase.database().ref('/Users/').push({
name: name,
age: age,
address : address
});
resetInputs();
});
</script>
</body>
</html>
Ask any question that you have in the contact section. Thank you!